The WebSocket
protocol, described in the specification RFC 6455 provides a way to exchange data between browser and server via a persistent connection. The data can be passed in both directions as “packets”, without breaking the connection and additional HTTP-requests.
- Socket Emit Cheat Sheet
- Socket Emit Cheat Sheet Pdf
- Socket Emit Cheat Sheet Download
- Socket Emit Cheat Sheet Printable

WebSocket is especially great for services that require continuous data exchange, e.g. online games, real-time trading systems and so on.
Get code examples like 'emit cheatsheet' instantly right from your google search results with the Grepper Chrome Extension.
A simple example
To open a websocket connection, we need to create new WebSocket
using the special protocol ws
in the url:
- Codota search - find any JavaScript module, class or function.
- Emit Takes the event you want called and what you want to send with it, like a message. On Takes an event name and a callback function with what do do when the event is triggered. Takes the connection and disconnect events by default but you can add custom events. Broadcast.emit Sends to every user but the sender. Sending and Rendering.
- Last Updated December 22nd, 2018 This tutorial was written using Python 3.6.Some of the code used is not compatible with version 2. In this tutorial we’ll be exploring how one can create a socket.io based webserver in Python using the socketio module.
There’s also encrypted wss://
protocol. It’s like HTTPS for websockets.
The wss://
protocol is not only encrypted, but also more reliable.
That’s because ws://
data is not encrypted, visible for any intermediary. Old proxy servers do not know about WebSocket, they may see “strange” headers and abort the connection.
On the other hand, wss://
is WebSocket over TLS, (same as HTTPS is HTTP over TLS), the transport security layer encrypts the data at sender and decrypts at the receiver. So data packets are passed encrypted through proxies. They can’t see what’s inside and let them through.
Once the socket is created, we should listen to events on it. There are totally 4 events:
open
– connection established,message
– data received,error
– websocket error,close
– connection closed.
…And if we’d like to send something, then socket.send(data)
will do that.
Here’s an example:
For demo purposes, there’s a small server server.js written in Node.js, for the example above, running. It responds with “Hello from server, John”, then waits 5 seconds and closes the connection.
So you’ll see events open
→ message
→ close
.
That’s actually it, we can talk WebSocket already. Quite simple, isn’t it?
Now let’s talk more in-depth.
Opening a websocket
When new WebSocket(url)
is created, it starts connecting immediately.
During the connection the browser (using headers) asks the server: “Do you support Websocket?” And if the server replies “yes”, then the talk continues in WebSocket protocol, which is not HTTP at all.
Here’s an example of browser headers for request made by new WebSocket('wss://javascript.info/chat')
.
Origin
– the origin of the client page, e.g.https://javascript.info
. WebSocket objects are cross-origin by nature. There are no special headers or other limitations. Old servers are unable to handle WebSocket anyway, so there are no compatibility issues. ButOrigin
header is important, as it allows the server to decide whether or not to talk WebSocket with this website.Connection: Upgrade
– signals that the client would like to change the protocol.Upgrade: websocket
– the requested protocol is “websocket”.Sec-WebSocket-Key
– a random browser-generated key for security.Sec-WebSocket-Version
– WebSocket protocol version, 13 is the current one.
We can’t use XMLHttpRequest
or fetch
to make this kind of HTTP-request, because JavaScript is not allowed to set these headers.
If the server agrees to switch to WebSocket, it should send code 101 response:
Here Sec-WebSocket-Accept
is Sec-WebSocket-Key
, recoded using a special algorithm. The browser uses it to make sure that the response corresponds to the request.
Afterwards, the data is transfered using WebSocket protocol, we’ll see its structure (“frames”) soon. And that’s not HTTP at all.
Extensions and subprotocols
There may be additional headers Sec-WebSocket-Extensions
and Sec-WebSocket-Protocol
that describe extensions and subprotocols.
For instance:
Sec-WebSocket-Extensions: deflate-frame
means that the browser supports data compression. An extension is something related to transferring the data, functionality that extends WebSocket protocol. The headerSec-WebSocket-Extensions
is sent automatically by the browser, with the list of all extensions it supports.Sec-WebSocket-Protocol: soap, wamp
means that we’d like to transfer not just any data, but the data in SOAP or WAMP (“The WebSocket Application Messaging Protocol”) protocols. WebSocket subprotocols are registered in the IANA catalogue. So, this header describes data formats that we’re going to use.This optional header is set using the second parameter of
new WebSocket
. That’s the array of subprotocols, e.g. if we’d like to use SOAP or WAMP:
The server should respond with a list of protocols and extensions that it agrees to use.
Adobe master collection cs6 for mac rutracker. For example, the request:
Response:
Here the server responds that it supports the extension “deflate-frame”, and only SOAP of the requested subprotocols.
Data transfer
WebSocket communication consists of “frames” – data fragments, that can be sent from either side, and can be of several kinds:
- “text frames” – contain text data that parties send to each other.
- “binary data frames” – contain binary data that parties send to each other.
- “ping/pong frames” are used to check the connection, sent from the server, the browser responds to these automatically.
- there’s also “connection close frame” and a few other service frames.
In the browser, we directly work only with text or binary frames.
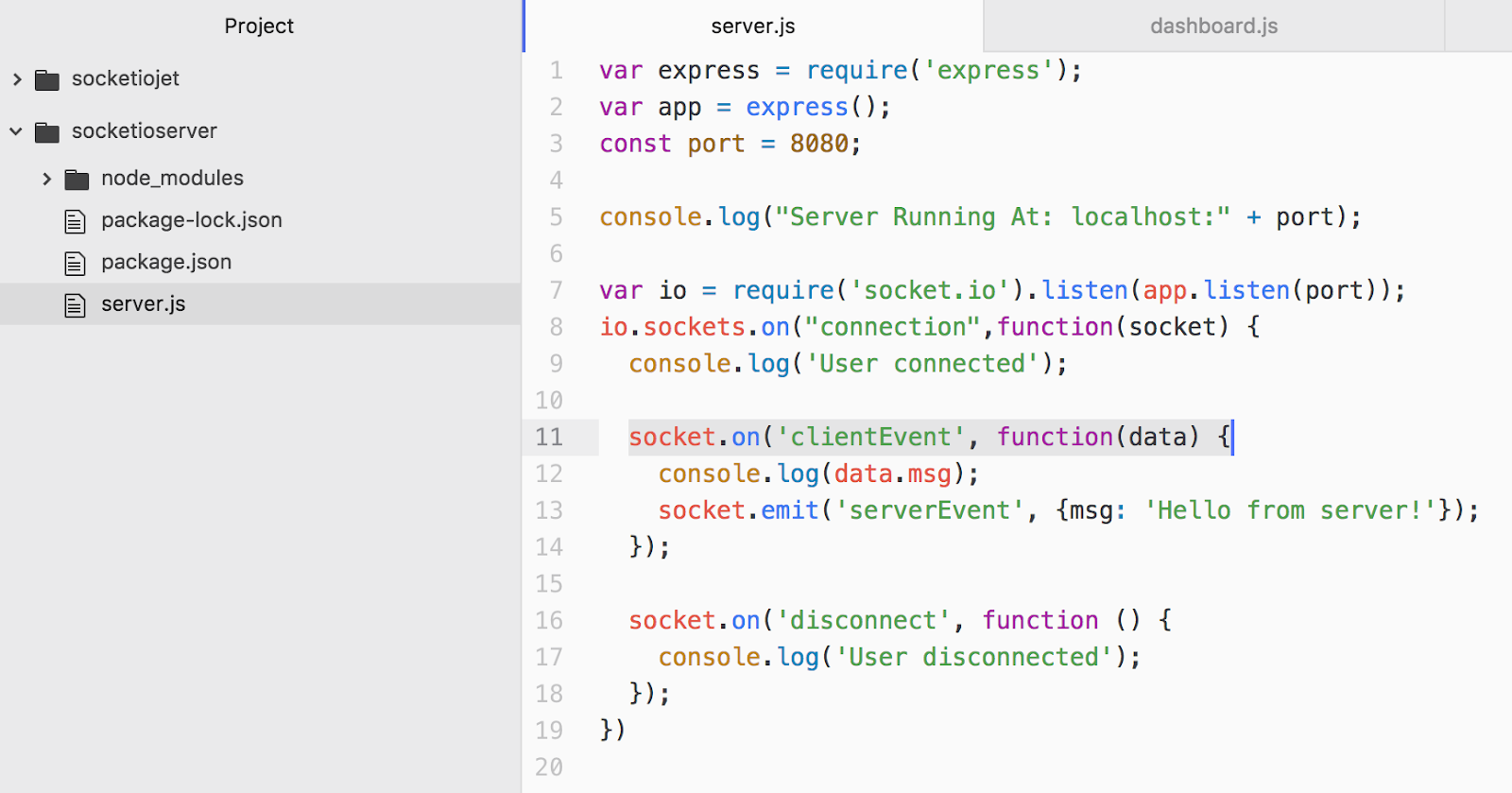
WebSocket .send()
method can send either text or binary data.
A call socket.send(body)
allows body
in string or a binary format, including Blob
, ArrayBuffer
, etc. No settings required: just send it out in any format.
When we receive the data, text always comes as string. And for binary data, we can choose between Blob
and ArrayBuffer
formats.
That’s set by socket.binaryType
property, it’s 'blob'
by default, so binary data comes as Blob
objects.
Blob is a high-level binary object, it directly integrates with <a>
, <img>
and other tags, so that’s a sane default. But for binary processing, to access individual data bytes, we can change it to 'arraybuffer'
:
Rate limiting
Imagine, our app is generating a lot of data to send. But the user has a slow network connection, maybe on a mobile internet, outside of a city.
We can call socket.send(data)
again and again. But the data will be buffered (stored) in memory and sent out only as fast as network speed allows.
The socket.bufferedAmount
property stores how many bytes remain buffered at this moment, waiting to be sent over the network.
We can examine it to see whether the socket is actually available for transmission.
Connection close
Normally, when a party wants to close the connection (both browser and server have equal rights), they send a “connection close frame” with a numeric code and a textual reason.
The method for that is:
code
is a special WebSocket closing code (optional)reason
is a string that describes the reason of closing (optional)
Then the other party in close
event handler gets the code and the reason, e.g.:
Most common code values:
1000
– the default, normal closure (used if nocode
supplied),1006
– no way to set such code manually, indicates that the connection was lost (no close frame).
There are other codes like:
1001
– the party is going away, e.g. server is shutting down, or a browser leaves the page,1009
– the message is too big to process,1011
– unexpected error on server,- …and so on.
The full list can be found in RFC6455, §7.4.1.
WebSocket codes are somewhat like HTTP codes, but different. In particular, any codes less than 1000
are reserved, there’ll be an error if we try to set such a code.
Connection state
To get connection state, additionally there’s socket.readyState
property with values: Microsoft whiteboard and teams.
0
– “CONNECTING”: the connection has not yet been established,1
– “OPEN”: communicating,2
– “CLOSING”: the connection is closing,3
– “CLOSED”: the connection is closed.
Chat example
Let’s review a chat example using browser WebSocket API and Node.js WebSocket module https://github.com/websockets/ws. We’ll pay the main attention to the client side, but the server is also simple.
HTML: we need a <form>
to send messages and a <div>
for incoming messages:
From JavaScript we want three things:
- Open the connection.
- On form submission –
socket.send(message)
for the message. - On incoming message – append it to
div#messages
.
Here’s the code:
Server-side code is a little bit beyond our scope. Here we’ll use Node.js, but you don’t have to. Other platforms also have their means to work with WebSocket.
The server-side algorithm will be:
- Create
clients = new Set()
– a set of sockets. - For each accepted websocket, add it to the set
clients.add(socket)
and setupmessage
event listener to get its messages. - When a message received: iterate over clients and send it to everyone.
- When a connection is closed:
clients.delete(socket)
.
Here’s the working example:
You can also download it (upper-right button in the iframe) and run locally. Just don’t forget to install Node.js and npm install ws
before running.
Summary
WebSocket is a modern way to have persistent browser-server connections.
- WebSockets don’t have cross-origin limitations.
- They are well-supported in browsers.
- Can send/receive strings and binary data.
The API is simple.
Methods:
socket.send(data)
,socket.close([code], [reason])
.
Events:
open
,message
,error
,close
.
WebSocket by itself does not include reconnection, authentication and many other high-level mechanisms. So there are client/server libraries for that, and it’s also possible to implement these capabilities manually.
Sometimes, to integrate WebSocket into existing project, people run WebSocket server in parallel with the main HTTP-server, and they share a single database. Requests to WebSocket use wss://ws.site.com
, a subdomain that leads to WebSocket server, while https://site.com
goes to the main HTTP-server.
Surely, other ways of integration are also possible.
Socket.io sending messages to individual clients
Emit cheatsheet, socket.emit('hello', 'can you hear me?', 1, 2, 'abc'); // sending to all clients except sender socket.broadcas. sending to individual socketid (private message) Sending message to all client works well but I want to send message to particular username. my server.js file looks like. socket.io and node.js to send message to
socket.io and node.js to send message to particular client, Try this: socket.on('pmessage', function (data) { // we tell the client to execute 'updatechat' with 2 parameters io.sockets.emit('pvt' To send a message to the particular client, we are must provide socket.id of that client to the server and at the server side socket.io takes care of delivering that message by using, socket.broadcast.to ('ID').emit ('send msg', {somedata : somedata_server}); For example,user3 want to send a message to user1.
Socket.io for simple chatting app - DEV, socket.emit('message', 'this is a test'); //sending to sender-client only 'for your eyes only'); //sending to individual socketid io.emit('message', Sending messages to certain clients with Socket.io I recently started playing with Socket.io and it’s really easy to get up and running. Just copy and paste their example and run the server. Open the page and open the console to see your messages being passed back and forth.
Socket.io send message to specific client
Send message to specific client with socket.io and node.js, Well you have to grab the client for that (surprise), you can either go the simple way: var io = io.listen(server); io.clients[sessionID].send(). Which may break, I I'm working with socket.io and node.js and until now it seems pretty good, but I don't know how to send a message from the server to an specific client, something like this: client.send(message, receiverSessionId) But neither the .send() nor the .broadcast() methods seem to supply my need.
Emit cheatsheet, hear me?', 1, 2, 'abc'); // sending to all clients except sender socket.broadcas. sending to a specific room in a specific namespace, including sender sending a message that might be dropped if the client is not ready to receive messages Sending messages to certain clients with Socket.io I recently started playing with Socket.io and it’s really easy to get up and running. Just copy and paste their example and run the server. Open the page and open the console to see your messages being passed back and forth.
Sending message to specific user with socket.io, To send a message to the particular client, we are must provide socket.id of that client to the server and at the server side socket.io takes care of delivering that message by using, socket.broadcast.to('ID'). emit( 'send msg', {somedata : somedata_server} ); For example,user3 want to send a message to user1. To send a message to the particular client, we are must provide socket.id of that client to the server and at the server side socket.io takes care of delivering that message by using, socket.broadcast.to ('ID').emit ('send msg', {somedata : somedata_server}); For example,user3 want to send a message to user1.
Socket.io emit to specific socket id
Socket Emit Cheat Sheet
Emit cheatsheet, sending to a specific room in a specific namespace, including sender io.of('myNamespace').to('room').emit('event', 'message'); // sending to individual socketid I want to sent data to one specific socket ID. We used to be able to do this in the older versions: io.sockets.socket(socketid).emit('message', 'for your eyes only'); How would I go about doing something similar in Socket.IO 1.0?
Sending message to a specific ID in Socket.IO 1.0, I want to sent data to one specific socket ID. We used to be able to do this in the older versions: io.sockets.socket(socketid).emit('message', // WARNING: `socket.to(socket.id).emit()` will NOT work, as it will send to everyone in the room // named `socket.id` but the sender. Please use the classic `socket.emit()` instead.
How can I send a message to a particular client with socket.io , Starting with socket.io + node.js, I know how to send a message locally and to broadcast io.to(socket.id).emit('event', data); How can we send message multiple time to a specific person or group in whatsapp using loop? Edit: If by id you're referring to the id value that socket.io assigns, not a value that you've assigned, you can get a socket with a specific id value using var socket = io.sockets.sockets['1981672396123987'];(above syntax tested using socket.io v0.7.9)
Socket io emit to multiple clients
For older version: You can store each client in an object as a property. Then you can lookup the socket based on the message: var basket = {}; io.sockets.on('connection', function (socket) { socket.on('register', function(data) { basket[data.nickname] = socket.id; }); socket.on('privmessage', function(data) { var to = basket[data.to]; io.sockets.socket(to).emit(data.msg); }); });
// sending to all clients in 'game1' and/or in 'game2' room, except sender socket.to('game1').to('game2').emit('nice game', 'let's play a game (too)'); // sending to all clients in 'game' room, including sender io.in('game').emit('big-announcement', 'the game will start soon'); // sending to all clients in namespace 'myNamespace', including sender
Socket Emit Cheat Sheet Pdf
socket.io - server emits multiple times. Socket.io client cannot emit message. 3. Socket IO The connection to ws://someAddress was interrupted while the page was
Socket io broadcast to specific users
socket.io - broadcast to certain users, There are two possibilites : 1) Each socket has its own unique ID stored in socket.id . If you know the ID of both users, then you can simply use There are two possibilites : 1) Each socket has its own unique ID stored in socket.id. If you know the ID of both users, then you can simply use. io.sockets[id].emit() 2) Define your own ID (for example user's name) and use. socket.join('priv/John'); in connection handler. Bash script visual studio code.
Emit cheatsheet, sending to the client socket.emit('hello', 'can you hear me?', 1, 2, 'abc'); will start soon'); // sending to a specific room in a specific namespace, including sender To send a message to the particular client, we are must provide socket.id of that client to the server and at the server side socket.io takes care of delivering that message by using, socket.broadcast.to ('ID').emit ( 'send msg', {somedata : somedata_server} ); For example,user3 want to send a message to user1.
Sending message to specific user with socket.io, Instead, we were broadcasting the messages and showing to the specific user that by AngularJs. But this article is truly based on Sending We can send the message to all the connected clients, to clients on a namespace and clients in a particular room. To broadcast an event to all the clients, we can use the io.sockets.emit method. Note − This will emit the event to ALL the connected clients (event the socket that might have fired this event). In this example, we will broadcast the number of connected clients to all the users.
Socket.io client not receiving messages from server
socket.io client not receiving messages from server, The function passed to .on is called for each socket to do the initialization (binding events etc), and socket refers to that current socket. This will I just cannot understand the reason why Client 2 does not receive the 'pop' message, I'm quite new to socket.io and node.js in general so some mechanics to me are still a bit obscure, so I apologize in advance for my noobness. :) cheers-k-
socket.io client not receiving · Issue #521 · socketio/socket.io · GitHub, But when I emit from server, the client does not seem to be receiving this What am i missing ? server: socket = io.listen(app); socket.sockets.on('connec NB: I also posted the same message in stackoverflow. If I get my Socket.IO server not receiving message from client. node.js,socket.io,mocha,bdd,expect.js. You need to change your code in two sides. First side, you will need to listen incoming socket connections on the socketIO object.
Why client are not receiving any data emitted? · Issue #1407 , here server.js 'use strict'; /** Module dependencies. / var http = require('http'), io = require('socket.io'), fs = require('fs'), passport Questions: I’m trying to implement a system with two clients one of them sends a message and the other one shall receive it. The figure below will explain it in a more visual way: So, the client 1 send the message to the server (and this works), the server receives a “push” message and emits
Socket io emit to room
Rooms, socket.broadcast.emit('broadcast', 'hello friends!'); // sending to all clients in 'game' room except sender socket.to('game').emit('nice game', 'let's play a game'); // sending to a specific room in a specific namespace, including sender io.of('myNamespace').to('room').emit('event', 'message'); // sending to individual socketid (private message) io.to(socketId).emit('hey', 'I just met you'); // WARNING: `socket.to(socket.id).emit()` will NOT work, as it will send to everyone in the room
Emit cheatsheet, emit() are the main two emit methods we use in Socket. io's Rooms (https://github.com/LearnBoost/socket.io/wiki/Rooms) Rooms allow simple partitioning of the connected clients. This allows events to be emitted with to subsets of the connected client list, and gives a simple method of managing them. You can emit to several rooms at the same time: io.to ('room1').to ('room2').to ('room3').emit ('some event'); In that case, an union is performed: every socket that is at least in one of the rooms will get the event once (even if the socket is in two or more rooms). You can also broadcast to a room from a given socket:
Socket.io rooms difference between broadcast.to and sockets.in , socket.broadcast.to('game').emit('message', 'nice game');. // sending to all clients in 'game' room(channel), include sender. io.in('game').emit('message', 'cool In socket.io, you usually use a specific syntax on the server side if you want to send a message to a specific room: io.to(room).emit('event', 'message');. But how would a client (what I mean is the socket.io-related code running in a browser) indicate that a message should go to a specific room?
Socket.io cheatsheet
io.on('connect', onConnect);function onConnect(socket){ // sending to the client socket.emit('hello', 'can you hear me?', 1, 2, 'abc'); // sending to all clients
Socket.io simple cheat sheet Raw. socket.js io. on ('connection', function (socket) {/* 'connection' is a special event fired on the server when any new connection is
Socket Emit Cheat Sheet Download
Socket.IO's 'Hello world' is a chat app in just a few lines of code. Document collaboration. Allow users to concurrently edit a document and see each other's changes.
Socket Emit Cheat Sheet Printable
More Articles
